Other API Architectures and Protocols - Examples and FAQs
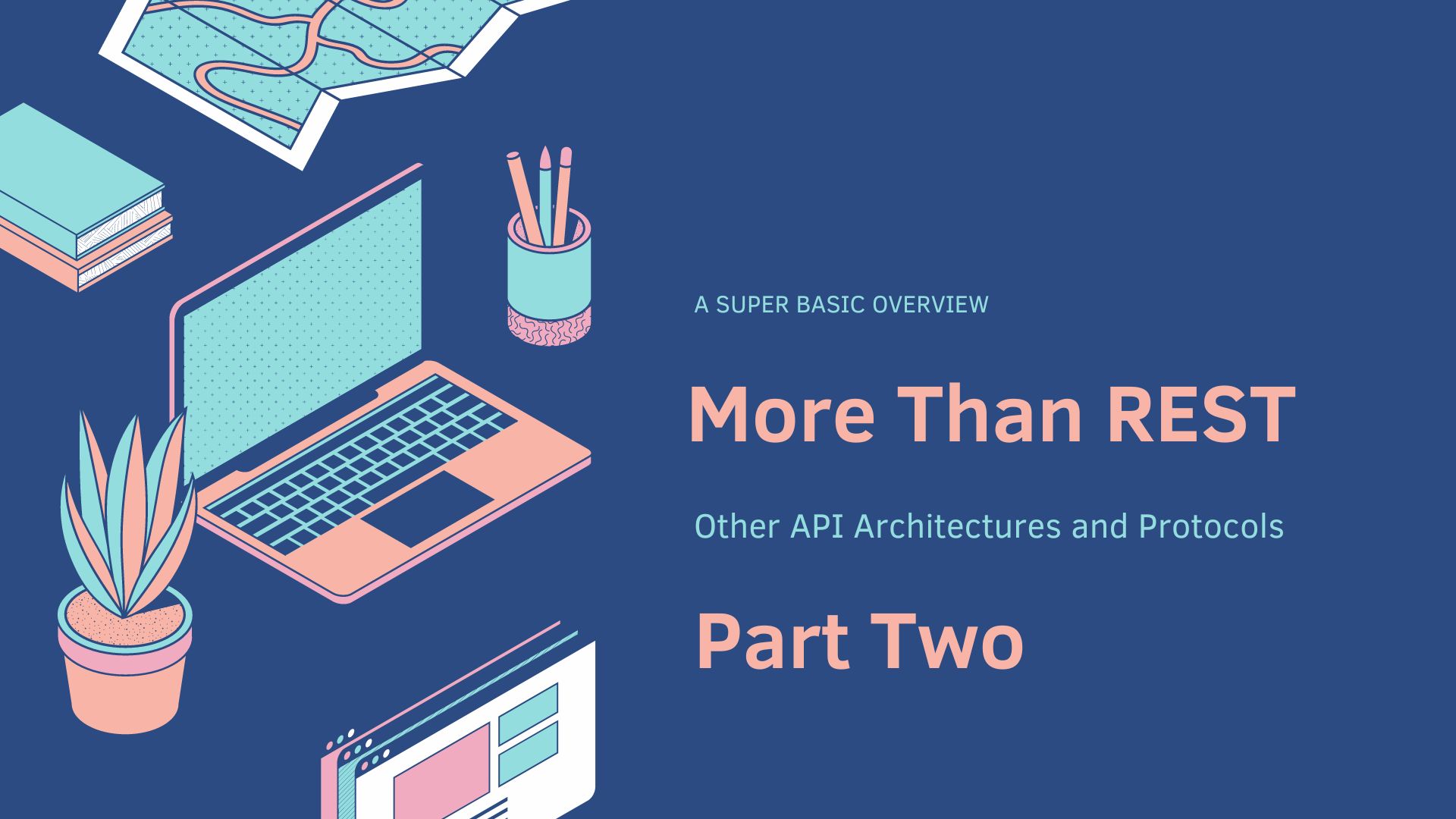
Info
Michelle Tabor
Mar 26, 2023 11:37:00 AM
Tags
Examples of Different Calls
RPC
Here is an example of an RPC API call using the JSON-RPC protocol:
Suppose we have a server application that provides a function to add two numbers, and a client application that wants to call this function remotely using an RPC API. The server application exposes an API endpoint at http://example.com/api, and the client application uses the curl command-line tool to send an RPC request to the server.
Here's an example of the RPC API call using JSON-RPC:
curl -X POST \ -H "Content-Type: application/json" \ -d '{"jsonrpc": "2.0", "method": "add", "params": [2, 3], "id": 1}' \ http://example.com/api
In this example, the -X POST option tells curl to send an HTTP POST request to the server. The -H "Content-Type: application/json" option sets the HTTP header to indicate that the request body is in JSON format. The -d option specifies the JSON-RPC request object, which includes the following fields:
- "jsonrpc": "2.0": specifies the JSON-RPC version.
- "method": "add": specifies the name of the remote function to be called.
- "params": [2, 3]: specifies the parameters to be passed to the remote function.
- "id": 1: specifies a unique identifier for the request.
When the server receives this request, it parses the JSON-RPC request object and executes the add function with the parameters 2 and 3. The server then sends a JSON-RPC response object back to the client, which includes the following fields:
- "jsonrpc": "2.0": specifies the JSON-RPC version.
- "result": 5: specifies the result of the add function.
- "id": 1: specifies the same identifier as the request.
The client can then parse the JSON-RPC response object and extract the result, which in this case is 5.
This is just a basic example, but RPC APIs can support more complex functions and data structures, as well as error handling and authentication mechanisms.
GraphQL
GraphQL is a query language and runtime for building APIs that was developed by Facebook. GraphQL APIs provide a more efficient and flexible way for client applications to retrieve and manipulate data from a server. With GraphQL, client applications can specify exactly what data they need and receive only that data, rather than having to parse and filter a large response from the server.
GraphQL APIs use a schema to define the data types and operations that are available to the client. The schema is a blueprint of the API that describes the types of data that can be retrieved, the relationships between those types, and the operations that can be performed on them. The schema is defined using the GraphQL schema definition language (SDL), which is a type-safe way of defining the API schema.
One of the key features of GraphQL APIs is the ability to specify the exact data that the client needs, using a single request. This is achieved through the use of queries, which are written in the GraphQL query language. A query specifies the fields and relationships of the data that the client needs, as well as any arguments or variables that are required to filter or paginate the data.
Here's an example of a GraphQL query:
query {
movie(id: "123") { title releaseYear director { name nationality } actors { name age gender } } }
In this example, the client is requesting data about a movie with the ID of "123". The query specifies the fields that the client needs, including the movie's title, release year, director's name and nationality, and the names, ages, and genders of the actors. The query is sent to the server as a single request, and the server returns a response that includes only the requested fields.
GraphQL APIs also support mutations, which are used to create, update, or delete data on the server. Mutations are written in a similar way to queries, but with different syntax and semantics. Mutations can specify the fields and arguments that are required to create, update, or delete data, and can also return a result that includes any changed data.
Overall, GraphQL APIs provide a powerful and flexible way for client applications to interact with a server, by allowing them to specify exactly what data they need and reducing the amount of data transferred between the client and server. However, GraphQL APIs can also be more complex to implement than other types of APIs, due to the need for a well-defined schema and query language.
SOAP
SOAP (Simple Object Access Protocol) is a messaging protocol that is used to exchange structured information between systems over the internet. SOAP APIs are built using the SOAP protocol and are used to expose web services that can be consumed by other applications or systems.
SOAP APIs use XML (eXtensible Markup Language) to define the message structure and data types that are exchanged between the client and server. SOAP messages are structured as envelopes that contain a header and a body. The header contains metadata about the message, such as the message ID and timestamp, while the body contains the payload data.
One of the key features of SOAP APIs is their support for a variety of data types, including complex objects, arrays, and references. This makes SOAP APIs suitable for exchanging large amounts of structured data between systems.
Another important feature of SOAP APIs is their support for built-in error handling and security mechanisms. SOAP APIs use a variety of standards to ensure the integrity and confidentiality of data being exchanged, such as WS-Security for authentication and encryption, and WS-Addressing for message routing and endpoint addressing.
Here's an example of a SOAP message:
<soap:Envelope xmlns:soap="http://schemas.xmlsoap.org/soap/envelope/"> <soap:Header> <AuthHeader xmlns="http://example.com/auth"> <Username>user123</Username> <Password>pass456</Password> </AuthHeader> </soap:Header> <soap:Body> <GetCustomerInfo xmlns="http://example.com/customer"> <CustomerId>12345</CustomerId> </GetCustomerInfo> </soap:Body> </soap:Envelope>
In this example, the SOAP message contains a header with an authentication token, and a body with a request to retrieve customer information for a specific customer ID. The message is sent to the server using the SOAP protocol, and the server processes the request and returns a response in a similar SOAP message format.
Overall, SOAP APIs are a powerful and flexible way to expose web services and exchange structured data between systems. However, SOAP APIs can be more complex to implement and consume than other types of APIs, due to their support for a wide range of data types and built-in error handling and security mechanisms.
Is it possible to integration different types of APIs together?
Yes, it is possible to integrate a REST API and a SOAP API. In fact, this is a common scenario in enterprise applications where different systems and technologies need to communicate with each other.
One approach to integrating a REST API and a SOAP API is to use a middleware layer that can translate between the two protocols. This middleware layer can receive requests from a REST API and translate them into SOAP requests that are sent to the SOAP API. Similarly, the middleware can receive responses from the SOAP API and translate them into REST responses that are sent back to the client.
Another approach to integrating a REST API and a SOAP API is to use a gateway or proxy that can act as a bridge between the two protocols. This gateway can sit between the client and the two APIs and translate requests and responses as needed. The gateway can also handle security and authentication concerns, such as translating between OAuth2 tokens used by the REST API and WS-Security tokens used by the SOAP API.
It's important to note that integrating a REST API and a SOAP API can be complex and requires careful planning and testing. It's important to understand the differences between the two protocols and the limitations of the middleware or gateway that is being used to translate between them. Additionally, it's important to ensure that the data being exchanged between the two APIs is properly transformed and validated to ensure consistency and data integrity.